This is a post that I’m “preserving” from the CoH Homecoming forums, because as history has taught us, nothing is forever, unless it’s constantly maintained. And since I have no control over what happens to the Homecoming forums, I’m going to preserve it here, because I think it’s worth preserving.
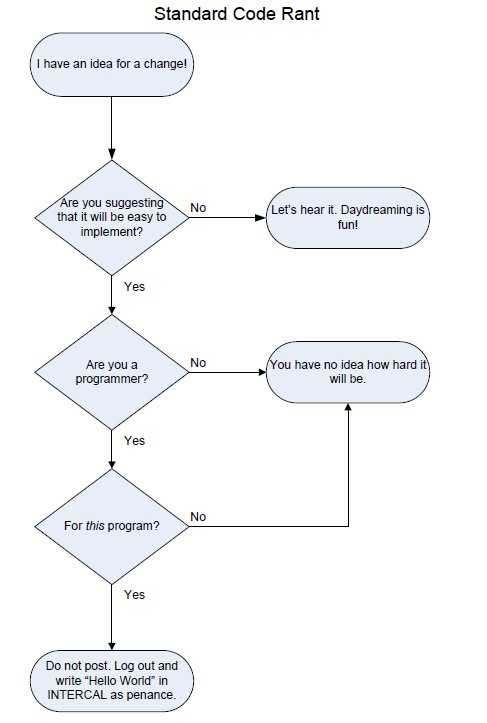
This was known on the original forums as The Standard Code Rant. I didn’t make it, someone else did. (Who? Anyone know? I’d love to talk to this genius!) Why do I do this? Why do I feel it’s important enough to keep doing it? Read on if you’d like to know.
I am a “professional” programmer by trade. Someone pays me money to type on my little keyboard in my little cubicle for 8+ hours a day. This is what I *DO*. Installed prominently in my cubicle, I have a piece of paper with the following text:
Westley’s Maxims
[*]Virtually ANYTHING is possible code, given enough time and resources.
[*]If you don’t know the existing code, or if a task has never been done before, there’s no way to accurately gauge how difficult it will be, until it is attempted.
[*]If the ONLY reason why you’re doing something a certain way is “because it’s always been done that way”, that is *NOT* an acceptable reason to keep doing it that way.
Why do I have this posted in my cubicle?
So that any time someone comes and asks me “can you do X”, I can point to #1.
So that any time someone suggests that something “should be easy, right?”, I can point to #2.
And so that any time I ask someone why they’re doing something “that way”, and they say “because it’s always been done that way”, I can point to #3.
It saves me a LOT of breath. The Standard Code Rant is essentially #2 stated in a more humorous and visual form. I don’t post it to be a COMPLETE dick, maybe just a partial dick, but no, I post it whenever I see “X should be easy” to raise AWARENESS that unless you’ve actually done something similar yourself, with THIS code base… you’re making a HUGE and gross assumption about that process.
Everything that you see on your computer screen is the result of literally MILLIONS of programming man(or woman)-hours of code work to make it appear on your screen, and make it useable to you. The modern programming languages that we have access to today are the results of MILLIONS more hours of programming work that had gone before us. Everything that I make or work on was built on the backs of giants, so that I don’t have to sit on my computer and figure out whether I should code my solution as “00010101” or as ” “00010110”. And thank GOD for that. I thank the tireless efforts of thousands or hundreds of thousands of programmers that came before me and laid the groundwork so that I can move forward and keep building more on top of what they’ve already given me.
Something that “sounds” easy to you, might be take a programmer anywhere from five minutes, to five months to accomplish, or anywhere in between. For example, below is a “Hello World” app that has a button that when you click on it, shows a message box that says “Hello World!”:
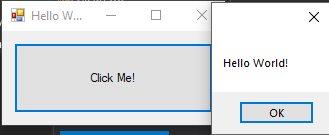
And below is the minimal required code to make that simple thing happen:
using System;
using System.Windows.Forms;
namespace Hello_World
{
static class Program
{
/// <summary>
/// The main entry point for the application.
/// </summary>
[sTAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Form1());
}
}
}
using System;
using System.Windows.Forms;
namespace Hello_World
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
MessageBox.Show(“Hello World!”);
}
}
}
Now, depending on your current level of computer know-how, that might all seem overwhelming and like Greek to you, or you might just be saying to yourself “is that it? so what?”. Well those saying “so what” aren’t realizing that for MY code to be even THAT simple, THOUSANDS of other lines of code behind the scenes had to be created by programmers that came before me that created all of the objects, methods, and other tools that I was able to use to make that program in the two minutes that it took me to throw it together. Someone had to MAKE the MessageBox class, someone had to MAKE the IDE (Integrated Development Environment) that allowed me to just plop an object down on a form in a GUI environment instead of making everything from scratch. NONE of this exists without someone going through the work and MAKING it exist. The wizards that came before me created the digital world, the digital land, digital air, digital waters, so that I could then populate it with digital trees and birds. But NONE of this would exist, if someone didn’t go through the work of making that world to begin with. *I* certainly couldn’t have made that world, I don’t have the skills necessary.
Now, let’s bring this back to the City of Heroes world. When City of Heroes was created, it was created in the language of C. Not C#, Not C++, but just plain old “regular” C. It was created in 1972. There WAS no easy “IDE” to use, there WERE no graphical tools that existed so that idiots like myself could plop pretty objects down onto other pretty objects. Hell, GUIs didn’t really even exists as a programming CONCEPT until a year after that language was originally envisioned, and wouldn’t become common until the mid 80s.
Now, why Cryptic chose to use regular C in 2003/whenever, I have no idea. But the fact is that they DID choose to use it. And, until someone rewrites the whole engine in a modern programming language, we’re stuck making everything the “hard way”. For example, here’s a snippet of code from source that draws a SINGLE one of the “quick access” selector buttons onscreen for you to click to change your chat channel by using just one of those little “letter buttons” above the chat window:
int drawSelectorButton(int * xp, int y, int z, float scale, int clr, Entity * e, int tip, int info, char * text, char * smallTex, char * largeTex)
{
AtlasTex * tex;
int hit = 0;
int x = *xp;
if( e->pl->chatSendChannel == info )
{
tex = atlasLoadTexture( largeTex );
z += 1;
}
else
{
tex = atlasLoadTexture( smallTex );
}
BuildCBox( &chatTip[tip].bounds, x – tex->width*scale/2, y – tex->height*scale, scale*tex->width, scale*tex->height );
setToolTip( &chatTip[tip], &chatTip[tip].bounds, text, 0, MENU_GAME, WDW_CHAT_BOX );
if( D_MOUSEHIT == drawGenericButton( tex, x – tex->width*scale/2, y – tex->height*scale, z, scale, clr, CLR_WHITE, e->pl->chatSendChannel != info ) )
{
e->pl->chatSendChannel = info;
sendChatChannel( e->pl->chatSendChannel, “” ); // won’t use buttons for user channels
hit = 1;
}
*xp += CHANNEL_SPACER*scale;
return hit;
}
And even THIS is just a SINGLE method that makes use of dozens of other variables and methods that are defined elsewhere in code. Just to DRAW one single teeny tiny pretty button.
Are you starting to get the complexity here? No? Well, take a look at the “How It Fits Together” page from OuroDev, and notice that there are FIFTEEN different unique servers/programs that all work together in conjunction with each other to bring the game that you know and love to your screen, and EACH of those elements contain within them HUNDREDS of methods, objects, and variables that are made up themselves of THOUSANDS of lines of code.
I did at one point have a graphic that showed how all of these pieces fit together so that you could have a graphical representation of the complexity, but I seem to have lost that at this time. If I find it again, I’ll modify my post and stick it here for reference.
So, when I post that “Standard Code Rant”, I hope you can understand that it’s NOT just to troll, but rather to try to foster an understanding of our own ignorance of the process. Make suggestions, as the Standard Code Rant states, it’s fun to dream! Dream big! Go nuts in your imagination. But, unless you are intimitely familiar with the codebase itself, do NOT presume to suggest how “easy” a suggestion would be to implement.
For if you do, I will be there, and I will be memeing. ALL over your thread.
And I’ll be doing it with this now, The Updated Standard Code Rant:
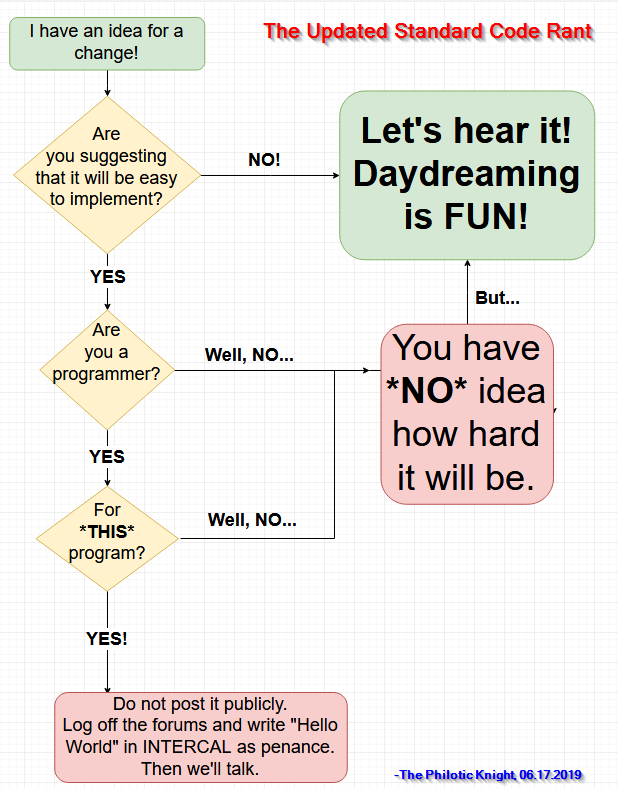